You’ve heard about AI chatbots, but have you explored the Ernie Bot API?
This powerful tool from Baidu is changing how businesses use AI. Ernie Bot API lets you add advanced language capabilities to your apps and services.
You can now use AI for tasks like market analysis and content creation, all through Baidu’s AI Cloud platform.
Want to try it out?
Since March 2023, invited users and businesses can access the API. Just register on the Baidu Ernie Bot platform to get started. The high demand speaks for itself – 30,000 companies applied for testing within an hour of launch. Now, the API handles 500 million queries daily.
Setting up is simple.
Install the SDK, grab your API key from your Baidu Research account, and you’re ready to go.
With Ernie Bot API, you’re unlocking a world of AI-powered possibilities for your business.
Overview of Ernie Bot API
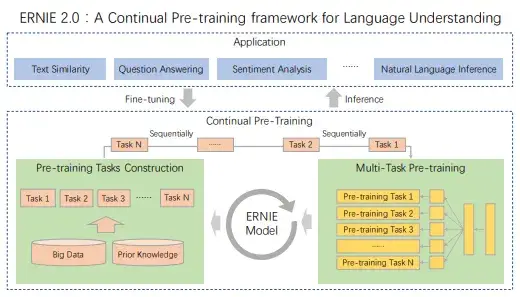
Ernie Bot API offers powerful natural language processing capabilities for businesses. It enables seamless integration of advanced AI features into various applications and services.
Core features
Ernie Bot can handle complex language tasks with ease. You can use it for text generation, sentiment analysis, and question answering.
One of its best features is its ability to understand context. This means it can give more accurate and relevant responses to user queries.
The API is highly scalable. It can handle 500 million daily queries, showing its power to manage large amounts of data.
Usage scenarios
You can use Ernie Bot API in many different ways.
It’s great for creating chatbots that can talk to customers in a natural way. This can improve your customer service and save time.
The API is also useful for content creation. It can help write articles, product descriptions, or social media posts.
You can use it for market analysis too. The API can process large amounts of text data to find trends and insights.
In education, you can use it to create smart tutoring systems. These can adapt to each student’s needs.
For developers, the API offers tools to build AI-powered apps and services quickly and easily.
Integration and setup
Setting up Baidu Ernie Bot API for your project is straightforward. You’ll need to complete authentication, meet system requirements, and follow installation steps. Let’s dive into the details.
Authentication process
To use Baidu Ernie Bot API, you need to get an API key. Here’s how:
- Sign up for a Baidu AI Cloud account.
- Go to the ERNIE Bot section.
- Apply for API access.
Once approved, you’ll get your unique API key. Keep it safe and don’t share it. You’ll use this key to authenticate your requests to the API.
Baidu Ernie Bot’s website offers a detailed guide on getting started.
System requirements
To use Baidu Ernie Bot API, your system needs:
- A stable internet connection
- Python 3.7 or higher
- At least 4GB of RAM
- 2GB of free disk space
Make sure your system meets these requirements before you start. This will ensure smooth operation of the API.
Installation guidelines
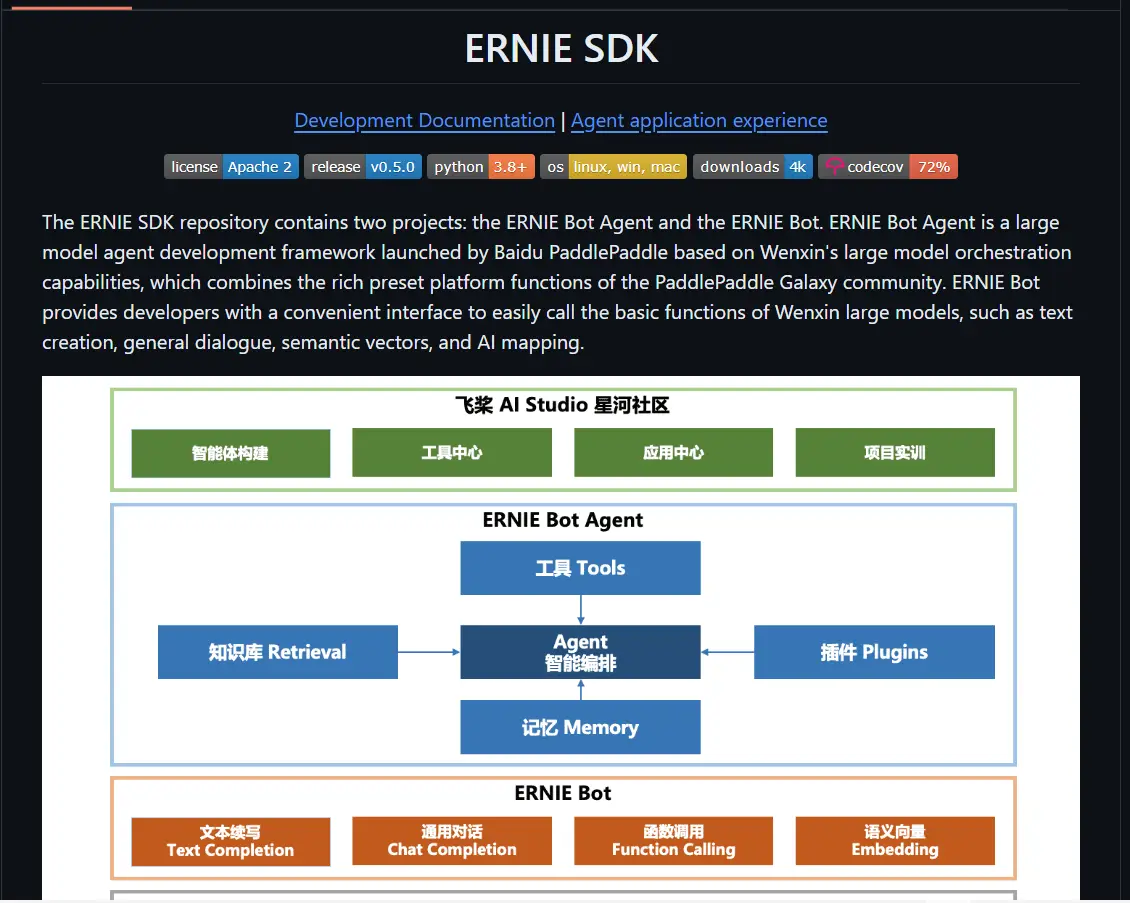
Follow these steps to install Baidu Ernie Bot API:
- Open your command prompt or terminal.
- Run this command:
pip install erniebot
- Wait for the installation to complete.
After installation, import the library in your Python script:
import erniebot
Set your API key as an environment variable:
export EB_ACCESS_TOKEN="your-api-key-here"
You’re now ready to start using Baidu Ernie Bot API in your projects. Remember to check the official documentation for the most up-to-date information on usage and features.
API reference
The ERNIE Bot API offers a robust set of tools for developers. You’ll find detailed information on endpoints, request formats, and error handling to help you integrate the API effectively and run Ernie latest models.
Endpoint structures
The ERNIE Bot API uses RESTful endpoints for various functions. You can access these endpoints through the base URL: https://api.baidu.com/ernie-bot/v1/
.
Key endpoints include:
/chat
: For natural language conversations/completions
: To generate text based on prompts/embeddings
: To create vector representations of text
Each endpoint requires specific parameters in the request body. For example, the /chat
endpoint needs a messages
array with user and system messages.
Request and response formats
Requests to the ERNIE Bot API use JSON format. You must include your API key in the headers for authentication.
A typical request structure:
{
"messages": [
{"role": "user", "content": "What is AI?"}
],
"model": "ernie-bot-3.5"
}
Responses are also in JSON format. They include the generated text, token usage, and other metadata.
Example response:
{
"id": "cmpl-123xyz",
"object": "chat.completion",
"created": 1684193535,
"result": "AI stands for Artificial Intelligence...",
"usage": {
"prompt_tokens": 10,
"completion_tokens": 31,
"total_tokens": 41
}
}
Error codes and handling
The API returns standard HTTP status codes to indicate success or failure. Common error codes include:
- 400: Bad Request
- 401: Unauthorized
- 429: Too Many Requests
- 500: Internal Server Error
Each error response includes a detailed message to help you troubleshoot. For example:
{
"error": {
"message": "Invalid API key provided",
"type": "invalid_request_error",
"code": "invalid_api_key"
}
}
To handle errors, check the status code of each response. Implement retry logic for temporary errors like rate limiting (429). For authentication issues, double-check your API key.
Example agent (translated GitHub version)
import asyncio
from erniebot_agent.agents import FunctionAgent
from erniebot_agent.chat_models import ERNIEBot
from erniebot_agent.tools import RemoteToolkit
async def main():
# Initialize the large language model
llm = ERNIEBot(model="ernie-3.5")
# Get the text-to-speech tool
tts_tool = RemoteToolkit.from_aistudio("texttospeech").get_tools()
# Create an agent, integrating the language model and tools
agent = FunctionAgent(llm=llm, tools=tts_tool)
# Have a general conversation with the agent
result = await agent.run("Hello, please introduce yourself")
print(result.text)
# The model returns something like:
# "Hello, I'm Wenxin Yiyan, a knowledge-enhanced large language model developed by Baidu. I can interact with people through dialogue, answer questions, assist in content creation, and efficiently help people obtain information, knowledge, and inspiration."
# Request the agent to automatically call the text-to-speech tool based on the input text
result = await agent.run("Convert the previous self-introduction to speech")
print(result.text)
# The model returns something like:
# "Based on your request, I have converted the self-introduction to an audio file. The file name is file-local-c70878b4-a3f6-11ee-95d0-506b4b225bd6. You can use any device or software that supports playing audio files to play this file. If you need further operations or have any other questions, please feel free to ask."
# Write the audio file output by the agent to test.wav, which can then be played
audio_file = result.steps[-1].output_files[0]
await audio_file.write_contents_to("./test.wav")
asyncio.run(main())
Best practices and security
When using the ERNIE Bot API, it’s crucial to focus on data protection and performance optimization. These factors help ensure a secure and efficient implementation of the API in your projects.
Data protection measures
To safeguard sensitive information when using the ERNIE Bot API, follow these key steps:
- Use encrypted connections (HTTPS) for all API requests.
- Store API keys securely. Never expose them in client-side code or public repositories.
- Implement rate limiting to prevent abuse.
- Regularly rotate API keys to reduce the risk of unauthorized access.
- Set up IP whitelisting to control which servers can access the API.
Apply strict access controls to limit who can use the API within your organization. Monitor API usage logs to detect any unusual activity that could signal a security breach.
Performance optimization
To get the best performance from the ERNIE Bot API, try these techniques:
- Use asynchronous requests when making multiple API calls.
- Implement caching for frequently requested data to reduce API load.
- Optimize your prompts to get more accurate and efficient responses.
- Batch requests when possible to reduce network overhead.
- Use compression for large payloads to speed up data transfer.
Monitor your API usage and response times. This helps you identify bottlenecks and optimize accordingly. Consider using a content delivery network (CDN) to reduce latency for users in different geographic locations.
Frequently asked questions
The ERNIE Bot API offers powerful capabilities for developers and businesses. Here are some common questions about integrating and using this AI technology in various applications.
How can I integrate the ERNIE Bot API into my application?
To integrate the ERNIE Bot API, you’ll need to register for an API key through Baidu’s developer platform.
Once you have your key, you can use the SDK to make API calls from your application. The integration process typically involves:
- Installing the ERNIE Bot SDK
- Configuring your API key
- Making API requests in your code
What are some example use cases for using the ERNIE Bot API?
The ERNIE Bot API can be used for many purposes:
- Customer support chatbots
- Content generation for websites or marketing materials
- Language translation services
- Sentiment analysis of customer feedback
- Question answering systems
ERNIE Bot can automate responses to common customer questions, helping reduce response times and provide 24/7 support.
How do I set up an account to use the ERNIE Bot API?
To set up an account:
- Visit the Baidu AI Cloud website
- Click on the sign-up or register button
- Fill out the required information
- Verify your email address
- Log in to access the developer dashboard
- Apply for API access if needed
Some features may need additional approval from Baidu before you can use them.
Are there any tutorials available for getting started with the ERNIE Bot API?
Yes, there are tutorials available to help you get started:
- Baidu provides official documentation and guides on their developer platform
- Third-party websites offer step-by-step tutorials for common tasks
- You can also find video tutorials on platforms like YouTube
GitHub repositories often contain code samples and example projects to help you learn.
What distinguishes the ERNIE Bot API from other language processing APIs?
ERNIE Bot has some unique features:
- It’s designed to understand and process Chinese language nuances very well
- The bot uses a knowledge-enhanced learning approach
- It can handle complex reasoning tasks
- ERNIE Bot is continuously updated with new capabilities
These features make it particularly strong for applications in Chinese markets.
What languages are supported by the ERNIE Bot API?
The ERNIE Bot API primarily supports:
- Chinese (both simplified and traditional)
Its strengths lie in Chinese language processing. It can handle English queries and tasks as well. Support for other languages may be added in future updates.